Written by Robert
Dunlop
Microsoft DirectX MVP |
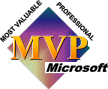 |

While supporting developer defined vertex formats using Flexible Vertex Formats, DirectX 7 also provided three pre-defined vertex types:
Vertex Type |
Description |
Contents |
D3DVERTEX |
Untransformed, Unlit Vertex Format |
3D Coordinate
Normal Vector
2D Texture Coordinate |
D3DLVERTEX |
Untransformed, Lit Vertex Format |
3D Coordinate
Diffuse Color
Specular Color
2D Texture Coordinate |
D3DTLVERTEX |
Transformed, Lit Vertex Format |
3D Coordinate
Reciprocal of Homogenous W
Diffuse Color
Specular Color
2D Texture Coordinate |
Under the DirectX 8 SDK, however, there are no predefined vertex types. While this is meant to encourage using your own vertex definitions that best suit your needs, this may seem a bit daunting at first - especially if you are porting an application from DX 7 that uses the pre-defined types.
To this end, below I have provided source code that can be used to provide support for the DX 7 vertex types. Similar definitions can be found in the DX8 C++ documentation under "Legacy FVF Format". However, the definitions below are written to easily compile with code previously written under DX 7.
Feel free to use these templates to aid in porting applications from previous versions, as well as sample templates that can be modified for your own vertex types.

D3DVERTEX Definition
#define D3DFVF_VERTEX D3DFVF_XYZ|D3DFVF_NORMAL|D3DFVF_TEX1
typedef struct _D3DVERTEX {
union {
float x;
float dvX;
}
union {
float y;
float dvY;
}
union {
float z;
float dvZ;
}
union {
float nx;
float dvNX;
}
union {
float ny;
float dvNY;
}
union {
float nz;
float dvNZ;
}
union {
float tu;
float dvTU;
}
union {
float tv;
float dvTV;
}
_D3DVERTEX() { }
_D3DVERTEX(const D3DVECTOR& v,const D3DVECTOR &n,float _tu, float _tv)
{ x = v.x; y = v.y; z = v.z;
nx = n.x; ny = n.y; nz = n.z;
tu = _tu; tv = _tv;
}
} D3DVERTEX, *LPD3DVERTEX;

D3DLVERTEX Definition
Note that the definition of a pre-lit vertex format in DX 8 does not require a DWORD to be inserted for alignment, as was required in DirectX 7.
#define D3DFVF_LVERTEX D3DFVF_XYZ|D3DFVF_DIFFUSE|D3DFVF_SPECULAR|D3DFVF_TEX1
typedef struct _D3DLVERTEX {
union {
float x;
float dvX;
}
union {
float y;
float dvY;
}
union {
float z;
float dvZ;
}
union {
D3DCOLOR color;
D3DCOLOR dcColor;
}
union {
D3DCOLOR specular;
D3DCOLOR dcSpecular;
}
union {
float tu;
float dvTU;
}
union {
float tv;
float dvTV;
}
_D3DLVERTEX() { }
_D3DLVERTEX(const D3DVECTOR& v,D3DCOLOR col,D3DCOLOR spec,float _tu, float _tv)
{ x = v.x; y = v.y; z = v.z;
color = col; specular = spec;
tu = _tu; tv = _tv;
}
} D3DLVERTEX, *LPD3DLVERTEX;

D3DTLVERTEX Definition
#define D3DTLFVF_VERTEX D3DFVF_XYZRHW|D3DFVF_DIFFUSE|D3DFVF_SPECULAR|D3DFVF_TEX1
typedef struct _D3DTLVERTEX {
union {
float x;
float dvX;
}
union {
float y;
float dvY;
}
union {
float z;
float dvZ;
}
union {
float rhw;
float dvRHW;
}
union {
D3DCOLOR color;
D3DCOLOR dcColor;
}
union {
D3DCOLOR specular;
D3DCOLOR dcSpecular;
}
union {
float tu;
float dvTU;
}
union {
float tv;
float dvTV;
}
_D3DTLVERTEX() { }
_D3DTLVERTEX(const D3DVECTOR& v,float w,D3DCOLOR col,D3DCOLOR spec, float _tu, float _tv)
{ x = v.x; y = v.y; z = v.z; rhw=w;
color = col; specular = spec;
tu = _tu; tv = _tv;
}
} D3DTLVERTEX, *LPD3DTLVERTEX;